If you have imported a CSV file into your notebook and use Pandas to view the dataframe you might find that the header of your spreadsheet is actually your first row of the dataframe.
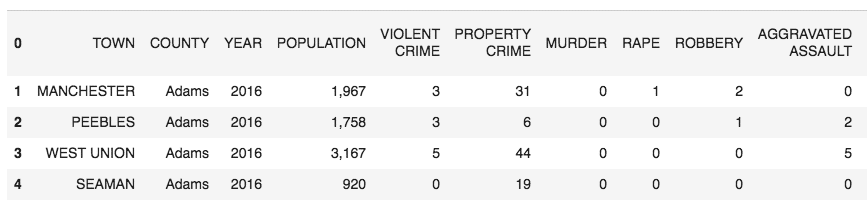
You can’t run analysis when your dataframe looks like the above. What you need is the first row to be your header and there is a simple way to do this in your notebook. First pull in your data:
#Convert to a DataFrame and render. import pandas as pd #Save the dataset in a variable df = pd.DataFrame.from_records(rows) # Lets see the 5 first rows of the dataset df.head()
Then, run the next bit of code:
# Create a new variable called 'new_header' from the first row of
# the dataset
# This calls the first row for the header new_header = df.iloc[0]
# take the rest of your data minus the header row df = df[1:]
# set the header row as the df header df.columns = new_header
# Lets see the 5 first rows of the new dataset df.head()